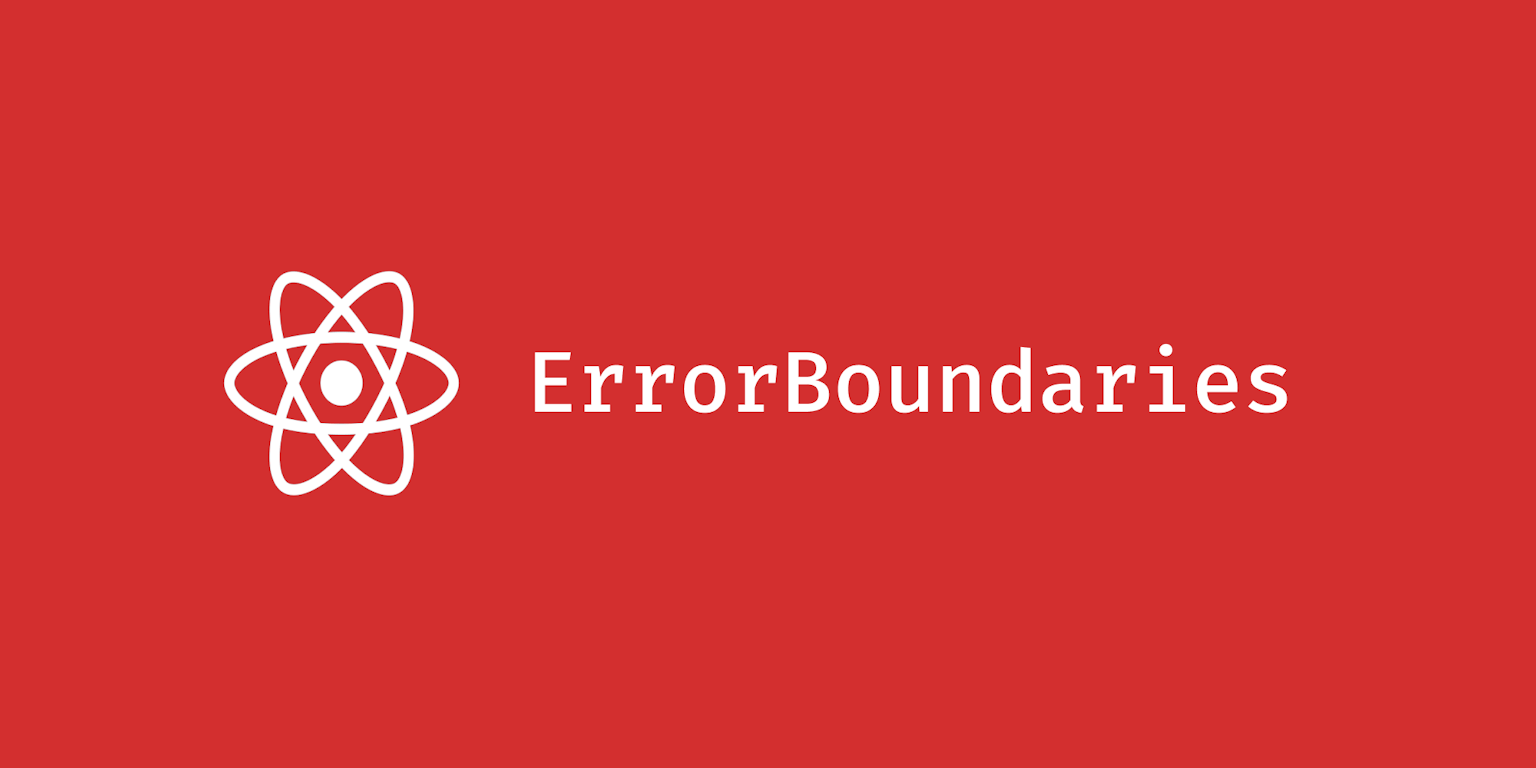
Managing React-Native crashes with Error Boundaries
2 min readReact 16 released a new concept called Error Boundary. This concept introduces a new way to catch JavaScript errors ๐ in a React project.
In this post I'm going to explain why it's important and how you can use error boundaries in a React-Native application to improve error resiliency, so let's get into it! ๐จโ๐ป
Why you should use them ?
According to the official React docs ๐:
As of React 16, errors that were not caught by any error boundary will result in unmounting of the whole React component tree ๐ฑ.
Unmounting the whole React component tree, means that if you don't catch errors at all the user will see an empty white screen ๐ฅ. Most of the time without having any feedback. This is not a great UX โ, fortunately you can fix this by using Error Boundaries โ .
How to use Error Boundaries
To benefit from Error Boundaries, we'll have to create a stateful component that will use the following lifecycle methods โป๏ธ:
getDerivedStateFromError
: This method is going to update the component state to display a fallback UI.componentDidCatch
: This method should be used to log the error to an external service.
So let's create the component that will catch errors in our application:
class ErrorBoundary extends React.Component {
state = { hasError: false }
static getDerivedStateFromError(error) {
return { hasError: true }
}
componentDidCatch(error, info) {
logErrorToService(error, info.componentStack)
}
render() {
return this.state.hasError ? <FallbackComponent /> : this.props.children
}
}
Pretty simple right? With a few lines of code, you can catch errors on your React-Native app ๐
To use it, all you need to do now is to wrap it around any component that could throw an error.
const App = () => (
<ErrorBoundary>
<Children />
</ErrorBoundary>
)
This component will catch all the errors that are thrown by any of his children. A common thing is to use it at the top level of your application ๐ to catch anything without having to use it on every screen or route ๐
That's how our FallbackComponent
looks whenever an error is thrown by our application ๐
โ ๏ธ Error Boundaries only catch JavaScript errors, all the native crashes that your application might have are not handled.
Introducing react-native-error-boundary
Few months ago, I created a simple, flexible and reusable React-Native error boundary component. Take a look into it ๐ if you're thinking about adding error boundaries to your app!
Enjoyed the article? ๐